Generate Hmac Sha256 Key C
Does anyone have an example on how to create a HMAC code using C#?
To generate an HMAC key using SHA-256, I can issue the following command: openssl dgst -sha256 -hmac key -binary mac.bin I realised (eventually!) that the key is not supplied as a hex string (0a0b34e5. Etc.) but in a binary format. PBKDF2 (Password-Based Key Derivation Function #2), defined in PKCS #5, is an algorithm for deriving a random value from a password. The algorithms applies a pseudo-random function - SHA256 HMAC in this case - to the password along with a salt string and repeats the process multiple times to create a derived key (i.e., a hash). HMAC Generator / Tester Tool. Computes a Hash-based message authentication code (HMAC) using a secret key. A HMAC is a small set of data that helps authenticate the nature of message; it protects the integrity and the authenticity of the message. Static void Main var data = 'testtesttest'; var secretKey = 'secretkey'; // Initialize the keyed hash object using the secret key as the key HMACSHA256 hashObject = new HMACSHA256(Encoding.UTF8.GetBytes(secretKey)); // Computes the signature by hashing the salt with the secret key as the key var signature = hashObject.ComputeHash(Encoding.UTF8.GetBytes(data)); // Base 64 Encode var. First, enter the plain-text and the cryptographic key to generate the code. Then, you can use select the hash function you want to apply for hashing. The default is SHA-256. Then you can submit your request by clicking on the compute hash button to generate the HMAC authentication code for you.
public static string CreateHMAC(string apiKey, string apiSecret, string token, string payload)
{
string randomNumber = '1';
string timestamp = DateTime.Now.Millisecond.ToString();
string temp = apiKey + randomNumber + timestamp + token + payload;
return getHashSha256(temp);
}
public static string getHashSha256(string text)
{
byte[] bytes = Encoding.UTF8.GetBytes(text);
SHA256Managed hashstring = new SHA256Managed();
byte[] hash = hashstring.ComputeHash(bytes);
string hashString = string.Empty;
foreach (byte x in hash)
{
hashString += String.Format('{0:x2}', x);
}
return hashString;
}
Hi Sean,
Unfortunately, we do not have an example of generating HMAC using C#. Please refer to Docs & Sandbox header section for any of our API that explains the logic and provides example code to generate HMAC in PHP, Objective-C & Python.
In your code, make sure that-
1. You are using an epoch timestamp in milliseconds
2. You Compute HMAC SHA256 hash on the data param using the API secret below.
3. As a final step, calculate the BASE64 of the hash.
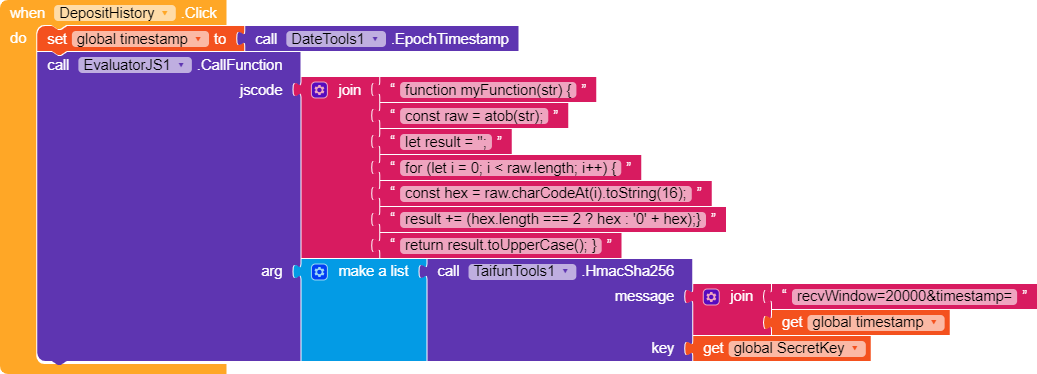
Regards,
Payeezy Team
Hi Sean,
You don't have to make the Hmac key from the code. It is very simple in two three steps to get the Hmac key.
First login to your Demo Payeezy account then open the terminals Shown on your Right side of window.
Then Click one terminal Then it will open a window, then select API Access window.
Then copy Copy Key Id and paste it on API_Key section in your code
Then below is the Hidden HMAC key hidden as Password, then click 'Generate New Key'.
It will gives you HMAC key. Copy it, Paste it in your code and Update the form.
Hi Mobeen,
What you mentioned relates to Payeezy Gateway but Sean is looking for is a way to integrate with the REST API.
Regards,
Payeezy Team.
Hi folks,
I have an example of this (and a full integration) available here:
https://github.com/clifton-io/Clifton.Payment
Specifically, the HMAC part can be found here:
https://github.com/clifton-io/Clifton.Payment/blob/a51ba4bd3de8b162a8a29471a93386dbea28900a/Clifton.Payment/Gateway/Payeezy/PayeezyGateway.cs#L42
/driver-booster-54-pro-serial-key-2018.html. Thanks
Brian
The code as it stands in the gitbug project didn't work for me. Here is a modified method that has been tested and works:
private string GenerateHMAC(string apiKey, string apiSecret, string token, int nonce, string currentTimestamp, string payload)
{
string message = apiKey + nonce.ToString() + currentTimestamp + token + payload;
HMAC hmacSha256 = new HMACSHA256(Encoding.UTF8.GetBytes(apiSecret));
byte[] hmacData = hmacSha256.ComputeHash(Encoding.UTF8.GetBytes(message));
string hex = BitConverter.ToString(hmacData);
hex = hex.Replace('-', ').ToLower();
byte[] hexArray = Encoding.UTF8.GetBytes(hex);
return Convert.ToBase64String(hexArray);
}
Hi blakeshadle5159,
Thanks for trying the code. I updated it, per your comment, and verified all the tests still pass:
https://github.com/clifton-io/Clifton.Payment/commit/d15b2fb0b05e775a78c038b54531d0aa5e075449
If the code is useful for you, please consider forking it and submitting pull requests if you discover any bugs or would like to add a feature
Brian,
Thank you for contributing.
Regards,
Generate Hmac Sha256 Key Commands
Payeezy team
Great Library. So easy to use. Thanks for making it.
I dont know if this is the library or payeezy stuff but I am getting a 401 access denied (Unathorized) error when I try to test out in my sandbox api a creditcard payment. I checked the keys and tokens and they are correct so not sure what I'm doing wrong.
21 Oct 2012I recently went through the processing of creating SDKs for an in house API. The API required signing every REST request with HMAC SHA256 signatures. Those signatures then needed to be converted to base64. Amazon S3 uses base64 strings for their hashes. There are some good reasons to use base64 encoding. See the stackOverflow question What is the use of base 64 encoding?
Below are some simplified HMAC SHA 256 solutions. They should all output qnR8UCqJggD55PohusaBNviGoOJ67HC6Btry4qXLVZc=
given the values of secret
and Message
. Take notice of the capital M. The hashed message is case sensitive.
Jump to an implementation:
Javascript HMAC SHA256
Run the code online with this jsfiddle.Dependent upon an open source js library called http://code.google.com/p/crypto-js/.
PHP HMAC SHA256
PHP has built in methods for hash_hmac (PHP 5) and base64_encode (PHP 4, PHP 5) resulting in nooutside dependencies. Say what you want about PHP but they have the cleanest code for this example.
Java HMAC SHA256
Dependent on Apache Commons Codec to encode in base64.
Groovy HMAC SHA256
It is mostly java code but there are some slight differences. Adapted from Dev Takeout - Groovy HMAC/SHA256 representation.
C# HMAC SHA256
Objective C and Cocoa HMAC SHA256
Most of the code required was for converting to bae64 and working the NSString and NSData data types.
Go programming language - Golang HMAC SHA256
Ruby HMAC SHA256
Requires openssl and base64.
Python (2.7) HMAC SHA256
Tested with Python 2.7.6. Also, be sure not to name your python demo script the same as one of the imported libraries.
Python (3.7) HMAC SHA256
Tested with Python 3.7.0. Also, be sure not to name your python demo script the same as one of the imported libraries. Thanks to @biswapanda.
Perl HMAC SHA256

See Digest::SHA documentation. By convention, the Digest modules do not pad their Base64 output. To fix this you can test the length of the hash and append equal signs '=' until it is the length is a multiple of 4. We will use a modulus function below.
Dart HMAC SHA256
Dependent upon the Dart crypto package.
Swift HMAC SHA256
I have not verified but see this stackOverflow post
Rust
Take a look at the alco/rust-digest repository for Rust (lang) guidance. I have not verified yet.
Powershell (Windows) HMAC SHA256
Mostly wrapping of .NET libraries but useful to see it in powershell's befuddling syntax. See code as gist
Shell (Bash etc) HMAC SHA256
Using openssl. Credit to @CzechJiri